In this blog we ‘ll learn about spring spel. spring spel stands for Spring Expression Language. We use SpEL in spring boot when we have to fetch any property from applications.properties or applications.yaml file.
While we use SpEL in spring boot we use # symbol with @value annotation. If you have already worked with spring boot application so it must be possible that you also aware of the $ that we use with @value annotation. @value(“${}”) this property is used to retrieve data from applications.properties or applications.yaml file, But it’s not part of the SpEL in spring boot.
And the key difference between # and $, when we use them for property injection is that # is load at run time whereas $ loads the property while application startup. So if you need any variable at the time of application startup use @value(“${}”) not @value(“#{}”)
SpEL (Spring Expression Language) uses # by enclosing it like #{} or @value(“#{}”) for property injection. Let’s see some of the example of SpEL in spring boot.
If you just want the code and don’t need to go through the explanation. You can directly jump to the code on github Code Github.
SpEL provide below support in spring boot application
- It provides support for conditional expressions
- Accessing Spring bean properties and methods
- It can access static constants and method of java
- It also used with spring annotations like @preAuthorize @postAuthorize etc.
Let’s do some coding now and see how this SpEL works in spring boot.
Logical Expression
@Value("#{5+5}")
private int sum;
Code language: CSS (css)

Calling static method of java
@Value("#{T(java.lang.Math).random() * 100}")
private double randomValue;
Code language: CSS (css)

We use T to call static method of java. Here we are using random() method which is a static method in java.lang.Math class. You can call any static method in the same way.
Accessing Bean class variable and methods
As we know using SpEL, we can access the variable or any method of our bean class. So to understand this first we ‘ll create a bean class where we define some variable or some method. Then we check how we can access the bean class variables and method using SpEL.
Let’s create a bean class first. In this bean class we are creating two variable along with their getter and also creating one method that is returning a string. You can create as per your requirement.
package com.javadream.SpELSpringBoot;
import org.springframework.stereotype.Component;
@Component("SpELBean")
public class SpELBean {
private String name = "Vasu Rajput";
private int age = 17;
//Getter method of variables
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String callMe() {
return "Java dream SpEL in Spring boot example";
}
}
Code language: PHP (php)
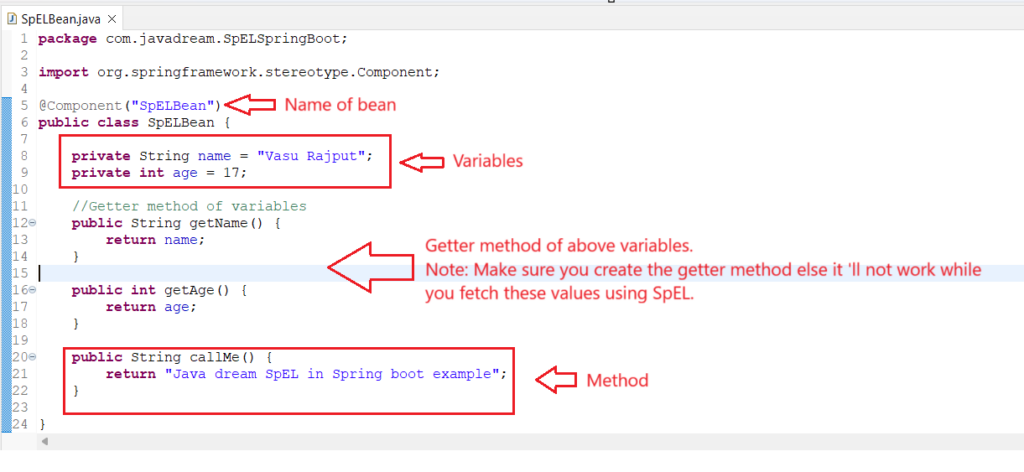
So till here we have our bean ready. Now look how we fetch these bean values using SpEL.
To Access name variable we ‘ll use the below expresssion
@Value("#{SpELBean.name}")
private String nameFromBean;
Code language: CSS (css)
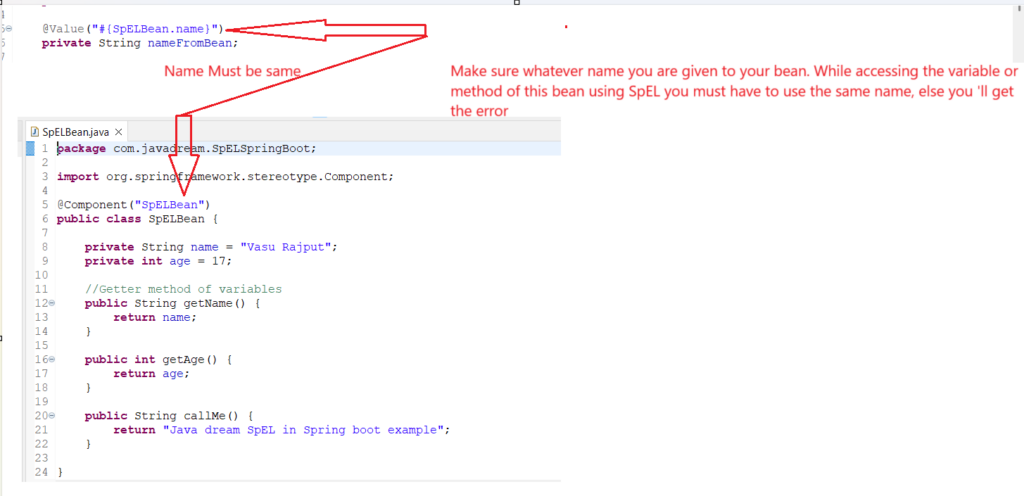
We can also use these variable for compression. Look for below example
@Value("#{SpELBean.name.equals('Vasu Rajput')}")
private boolean isEqual;
Code language: PHP (php)
In your bean class if name variable is Vasu Rajput then this return true else it return false. You can make this condition as per your requirement.
We can also make any conditional expression for the bean variable. For example look at below example.
@Value("#{SpELBean.age >= 18 ? 'valid For Voting' : 'Not Valid For Voting'}")
private String votingStatus;
Code language: JavaScript (javascript)
Here if in our bean class our age variable has value greater or equal to 18 then it ‘ll print valid for Voting else it ‘ll print Not Valid For Voting.
For calling bean class method using SpEL, Use the below expression.
@Value("#{SpELBean.callMe()}")
private String callBeanMethod;
Code language: CSS (css)
Creating Object using SpEL
We can also create object using SpEL. For example look at the below example.
@Value("#{new String('Hello Javadream. Example of SpEL in Spring Boot')}")
private String messageObj;
Code language: JavaScript (javascript)
The above expression create a new String object and when we print this messageObj variable, It ‘ll print the string that we defined in the expression.
Fetching value from applications.propertis or applications.yaml file
We can also fetch the values from applications.properties or applications.yaml file. For this we just have to define the environment key before the parameter value.
For example if my application.properties file has spring.application.name=SpELSpringBoot property. So to access it via SpEL our expression ‘ll be like below.
@Value("#{environment['spring.application.name']}")
private String applicationName;
Code language: CSS (css)
We have now some understanding of SpEL. Let’s create a project and try to test all these expressions there.
Project Structure:
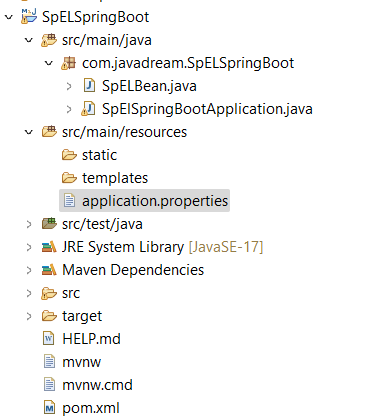
As mentioned above we create a simple bean class with some variable and methods. And in our main class we implements the CommandLineRunner interface. This interface provide a run method which execute after our application start. This method runs only once after all spring beans class have been initialized.
SpELBean.java
package com.javadream.SpELSpringBoot;
import org.springframework.stereotype.Component;
@Component("SpELBean")
public class SpELBean {
private String name = "Vasu Rajput";
private int age = 17;
//Getter method of variables
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String callMe() {
return "Java dream SpEL in Spring boot example";
}
}
Code language: PHP (php)
applications.properties
spring.application.name=SpELSpringBoot
SpElSpringBootApplication.java
package com.javadream.SpELSpringBoot;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import jakarta.annotation.PostConstruct;
@SpringBootApplication
public class SpElSpringBootApplication implements CommandLineRunner {
private static final Logger logger=LoggerFactory.getLogger(SpElSpringBootApplication.class);
@Value("#{5+5}")
private int sum;
@Value("#{T(java.lang.Math).random() * 100}")
private double randomValue;
@Value("#{SpELBean.name}")
private String nameFromBean;
@Value("#{SpELBean.name.equals('Vasu Rajput')}")
private boolean isEqual;
@Value("#{SpELBean.age >= 18 ? 'valid For Voting' : 'Not Valid For Voting'}")
private String votingStatus;
@Value("#{SpELBean.callMe()}")
private String callBeanMethod;
@Value("#{environment['spring.application.name']}")
private String applicationName;
@Value("#{new String('Hello Javadream. Example of SpEL in Spring Boot')}")
private String messageObj;
public static void main(String[] args) {
SpringApplication.run(SpElSpringBootApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
logger.info("sum: {}", sum);
logger.info("randomValue: {}", randomValue);
logger.info("nameFromBean: {}", nameFromBean);
logger.info("isEqual: {}", isEqual);
logger.info("applicationName: {}", applicationName);
logger.info("messageObj: {}", messageObj);
logger.info("votingStatus: {}", votingStatus);
logger.info("callBeanMethod: {}", callBeanMethod);
}
}
Code language: JavaScript (javascript)
If you see we are using all the SpEL expression that we explain above. And we are printing all these variables value inside run method of CommandLineRunner interface. So once you start your application in logs you can see values of these variables.
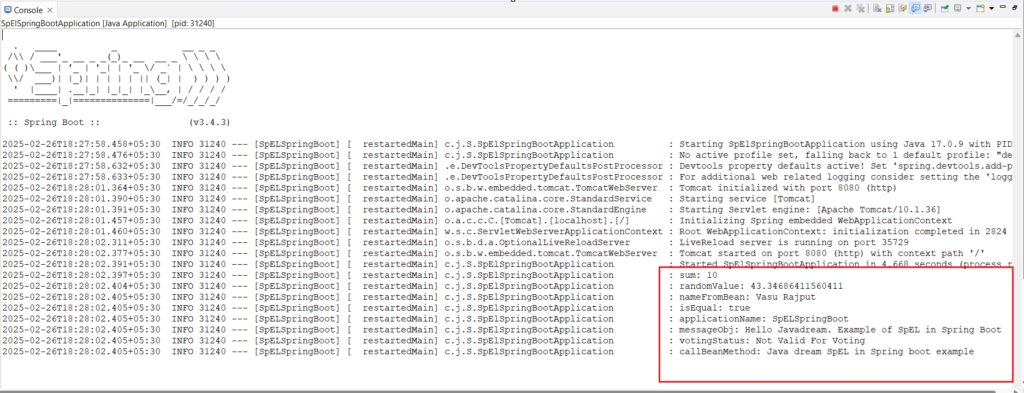