Spring boot integration with Twilio is the most interesting topic when we think about creating a spring boot application that uses SMS services. We know that Twilio provide the services for sending SMS, WhatsApp messages, Voice calls etc. It’s one of the best service provider in terms of sending SMS and Calls. If we want to make a application where we have to send the SMS to our clients than we can consider the Twilio as one of the best option.
If you just want to see the code only and do not want to go into the depth you can skip and go to the code directly using this link Go To Code
In this blog we ‘ll see how we can do the spring boot integration with Twilio for sending the SMS’s. It is very easy as Twilio provides it’s own SDK dependency. We can find the latest SDK dependency from maven repository (Maven Repository) and use it in our pom.xml file.
We ‘ll cover this spring boot integration with Twilio in below points.
- Setup you Twilio Account and setup a number that ‘ll be used for sending SMS.
- Create Spring boot application to use Twilio SMS services.
- Test the Spring boot application for sending SMS to client mobiles.
Setup Twilio Account
Visit the Twilio website on URL Twilio Account. A Sing up page ‘ll come up we just need to provide our details and continue. After signup you have to verify your email account and mobile number, after this Twilio provides a Recovery code. Once you done with all these step Twilio ask some question on how you want to use the Twilio service just fill that according to your need. Now you are all set to use Twilio. You can refer below screen shot of the described process.
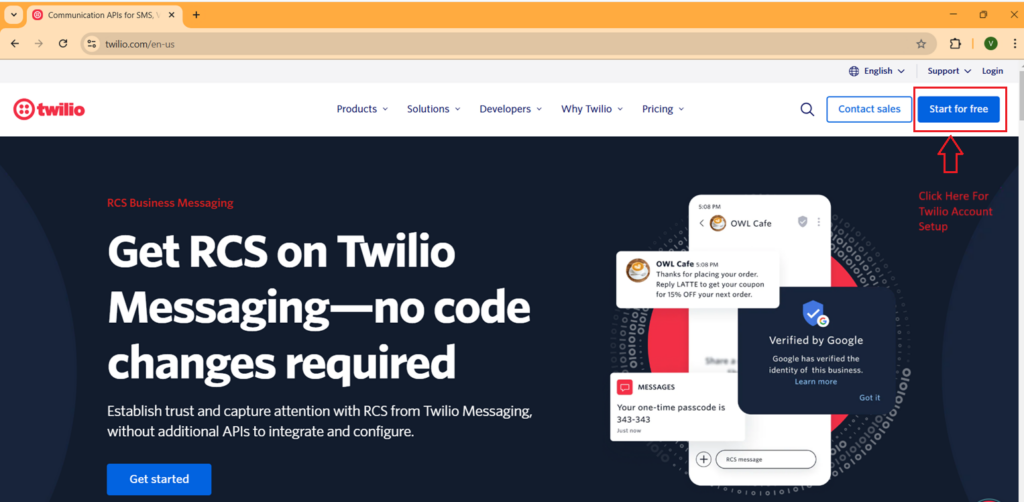
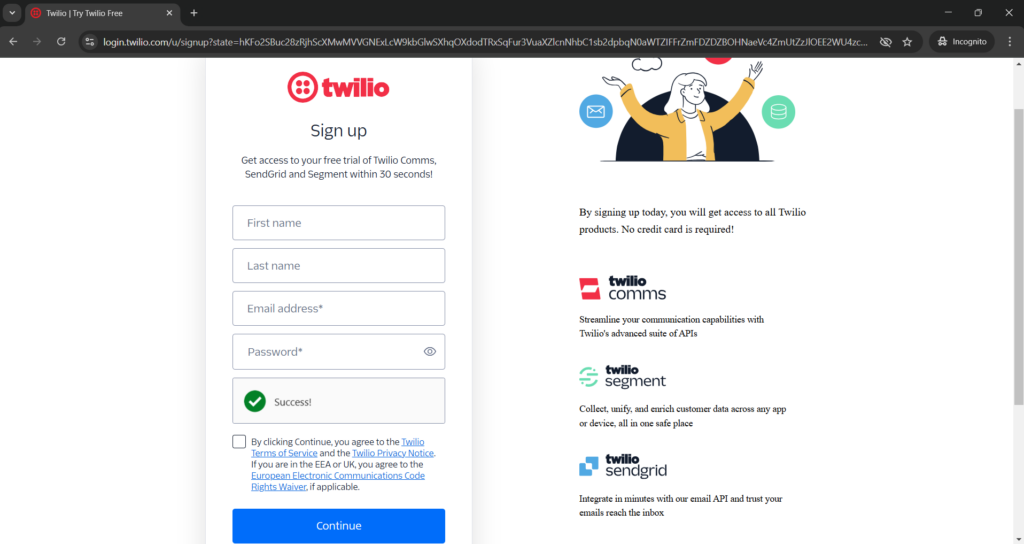

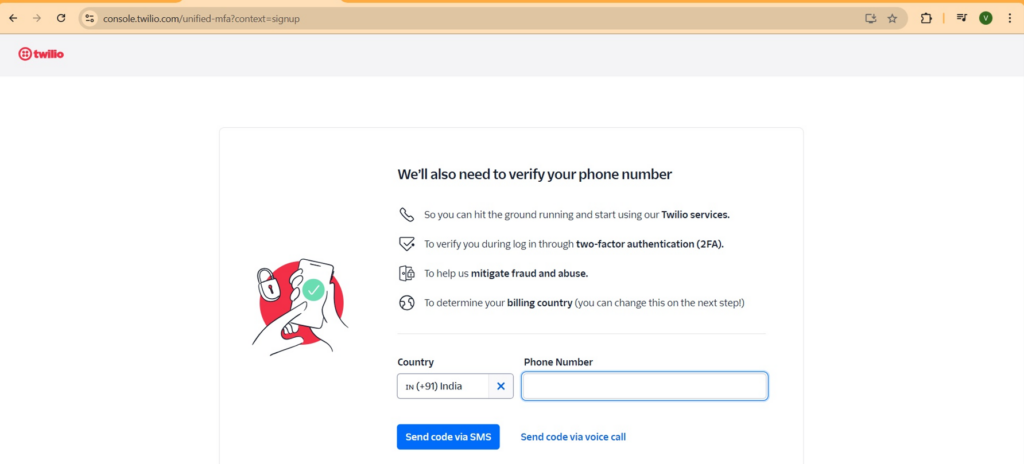
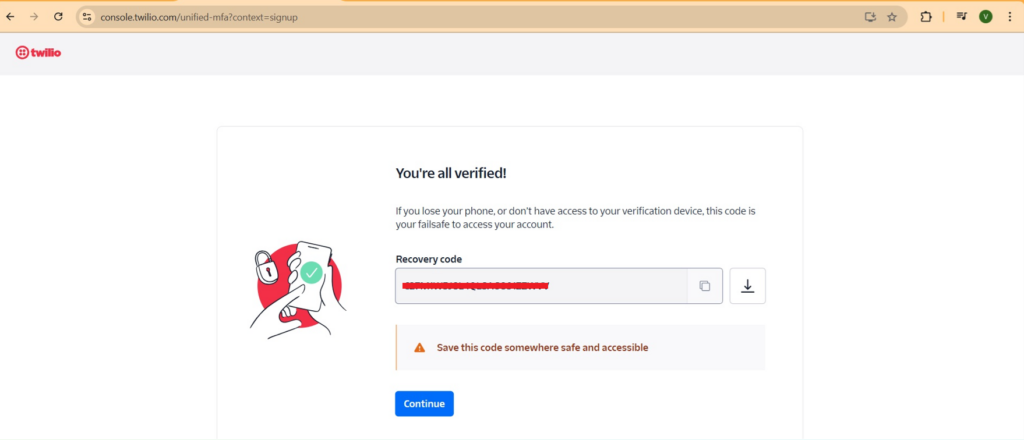
Setup Mobile Number
So you have your Twilio account setup. Now we need a Twilio number using which we can send the SMS to the clients. You can see this number in Active numbers tab on your dashboard.

Twilio Account SID and Auth Token
So we have got our Twilio number also. There are two more important things also that we have to collect from Twilio. We need the AccountSID and Auth Token.
Using these information Twilio mobile number, AccountSID, Auth Token we can integrate Twilio account to our Spring boot application. For getting this AccountSID and AuthToken go to your Twilio dashboard home screen and scroll down till bottom. On bottom you ‘ll get all these these details.

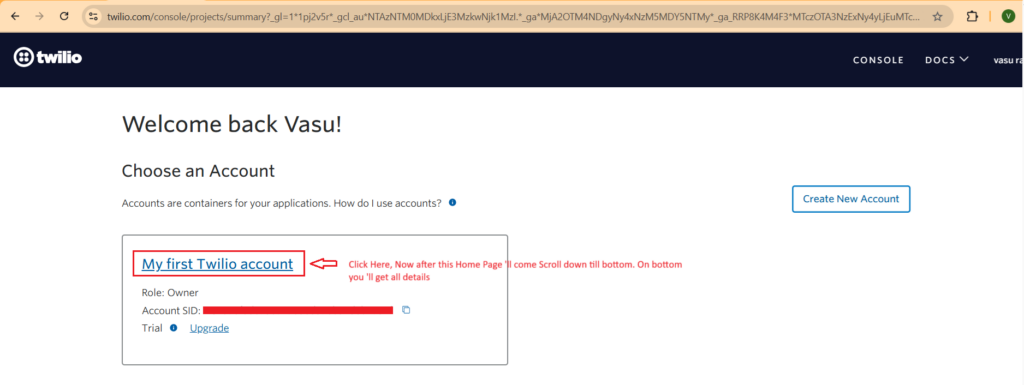

Spring boot integration with Twilio
We have successfully created our Twilio account. It’s time to integrate this account with Spring boot application for sending the SMS. As we told in the start it’s very easy to integrate because Twilio provides it’s own SDK. We can get this SDK dependency from here . Now let’s create a Spring boot application and start integrating Twilio account.
Project structure look like below for complete application that we ‘ll develop:
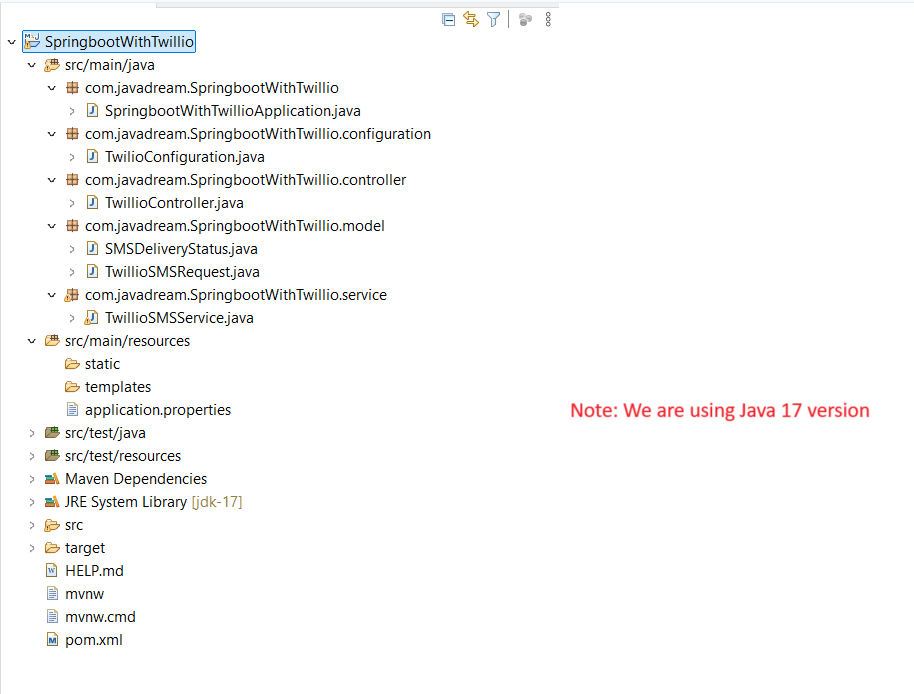
Go to the pom.xml file and add the below dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<!-- Dependency for Twillio -->
<dependency>
<groupId>com.twilio.sdk</groupId>
<artifactId>twilio</artifactId>
<version>10.6.7</version>
</dependency>
Code language: HTML, XML (xml)
If you remember on our Twilio dashboard we find the AccountSID, Auth Token and Twilio Phone number. We just need to copy that values and paste them in the below properties in your application.properties file. Follow the screenshot for your reference.
spring.application.name=SpringbootWithTwillio
twilio.account.sid=
twilio.auth.token=
twilio.phone.number=
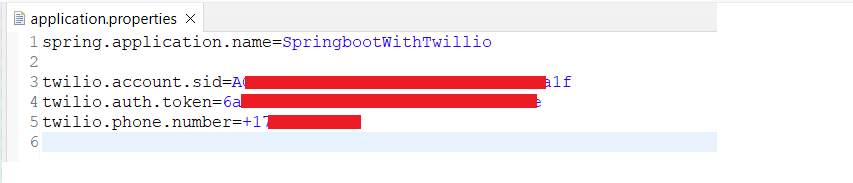
After setting up these properties, We have to initialize Twilio. For this we have to create a configuration class. We created a class with name TwilioConfiguration. Use the below code snippet:
package com.javadream.SpringbootWithTwillio.configuration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
import com.twilio.Twilio;
import jakarta.annotation.PostConstruct;
@Configuration
public class TwilioConfiguration {
private static final Logger logger = LoggerFactory.getLogger(TwilioConfiguration.class);
@Value("${twilio.account.sid}")
private String twillioAccountSID;
@Value("${twilio.auth.token}")
private String twillioAuthToken;
@PostConstruct
public void initializeTwillio() {
logger.info("Twillio intialization ...");
Twilio.init(twillioAccountSID, twillioAuthToken);
}
}
Code language: JavaScript (javascript)
Now we are totally ready. We just need to create a POST api that accept mobile number of the client and the message that we want to send. And need to write a service class where we put business logic to send SMS to the client. Follow the below code snippet for creating a POST api.
@PostMapping("/sendSMSMessage")
public String sendSMS(@RequestBody TwillioSMSRequest twillioSMSRequest) {
String sendSMSResponse = twillioSMSService.sendSMS(twillioSMSRequest.getToMobileNumber(),
twillioSMSRequest.getSmsBody());
return sendSMSResponse;
}
Code language: JavaScript (javascript)
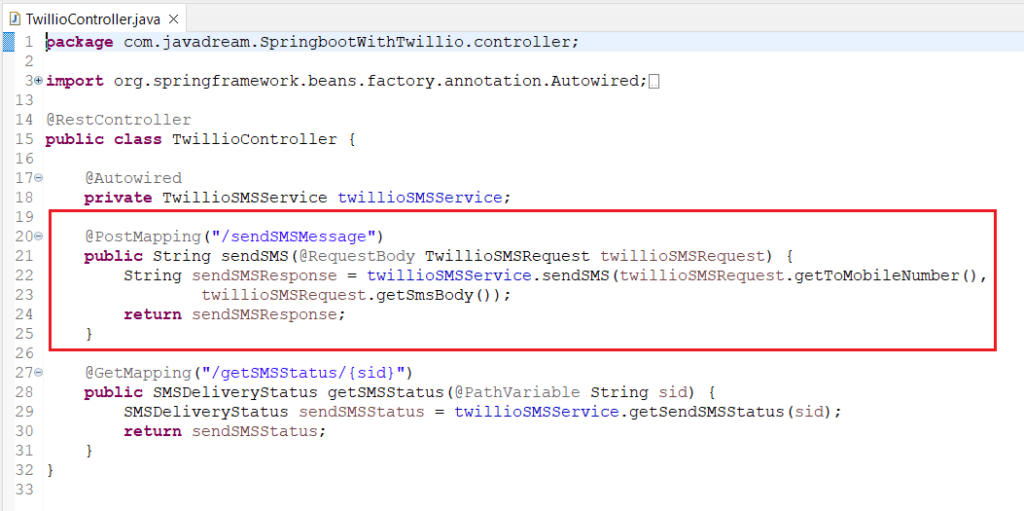
TwillioSMSRequest is the POJO class. As we know spring boot automatically convert json to the pojo class. Follow below code snippet for TwillioSMSRequest.
package com.javadream.SpringbootWithTwillio.model;
public class TwillioSMSRequest {
private String toMobileNumber;
private String smsBody;
public String getToMobileNumber() {
return toMobileNumber;
}
public void setToMobileNumber(String toMobileNumber) {
this.toMobileNumber = toMobileNumber;
}
public String getSmsBody() {
return smsBody;
}
public void setSmsBody(String smsBody) {
this.smsBody = smsBody;
}
}
Code language: JavaScript (javascript)
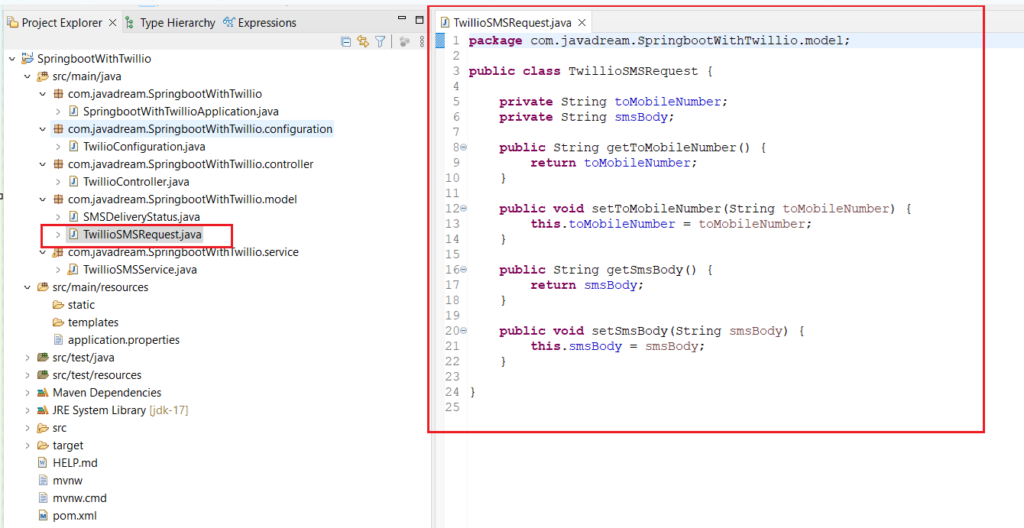
Now We need to create a service class that contains business logic to send SMS to the client. So for this we have created TwillioSMSService class. Follow the code snippet for this service class:
@Value("${twilio.phone.number}")
private String twilioPhoneNumber;
public String sendSMS(String toMobileNumber, String messageToSend) {
String sid;
try {
logger.info("Sending SMS to mobileNumber {}", toMobileNumber);
Message message = Message
.creator(new PhoneNumber(toMobileNumber), new PhoneNumber(twilioPhoneNumber), messageToSend)
.create();
sid = message.getSid();
} catch (Exception e) {
logger.error("Exception while sending SMS to mobileNumber: {} {}", toMobileNumber, e);
throw new RuntimeException("Failed to send SMS to mobileNumber: " + toMobileNumber);
}
return "SMS Send succesfully & sid is: " + sid;
}
Code language: JavaScript (javascript)
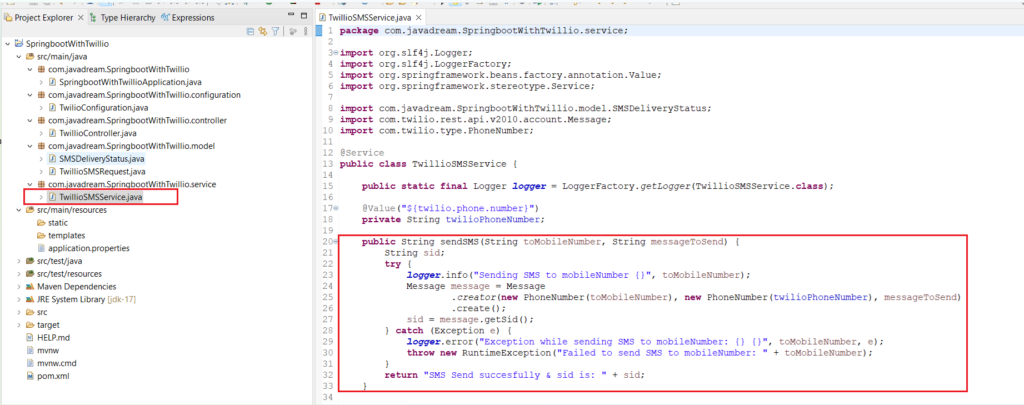
Testing
If you have follow till here, you are now ready to send the SMS to the client. Run your spring boot application and after that open your postman application and call this post api. After calling api just check the mobile for the given mobile number. You must receive the SMS. And on API response on postman application you ‘ll get a sid. This sid is used to get the information about the SMS delivery status, SMS cost and other details.
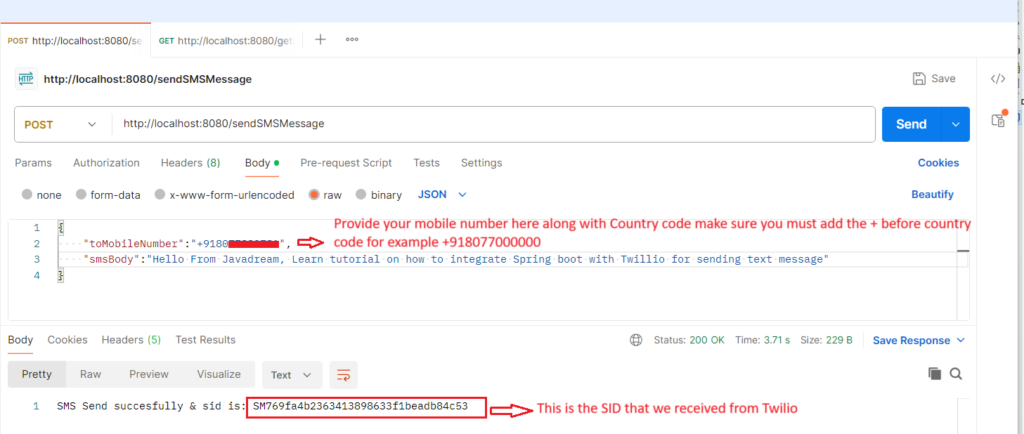
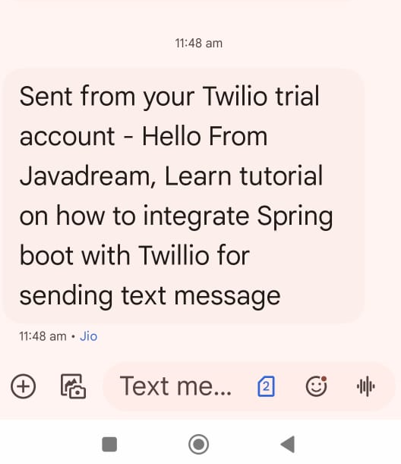
You see this Sent from your Twilio trial account in the start of message when you receive the SMS. This is because we are using the free trial of Twilio. Twilio attach this string in the start so no one can misuse the free trial account phone number. There is no way to get rid of this string until you are using free trial version. Once you use the paid number this string ‘ll remove and you can send your customized message to the client.
Delivery Status
We have successfully send the SMS to the client. But we have to check the delivery status of these SMS to make sure that our SMS has been delivered to client succesfully.
So there are Two ways using which you can check the status of the SMS.
- Using Twilio Dashboard
- Using Programmatic way
Using Twilio Dashboard
Using Twilio dashboard go to Monitor -> Messaging. You ‘ll see the delivery status there.
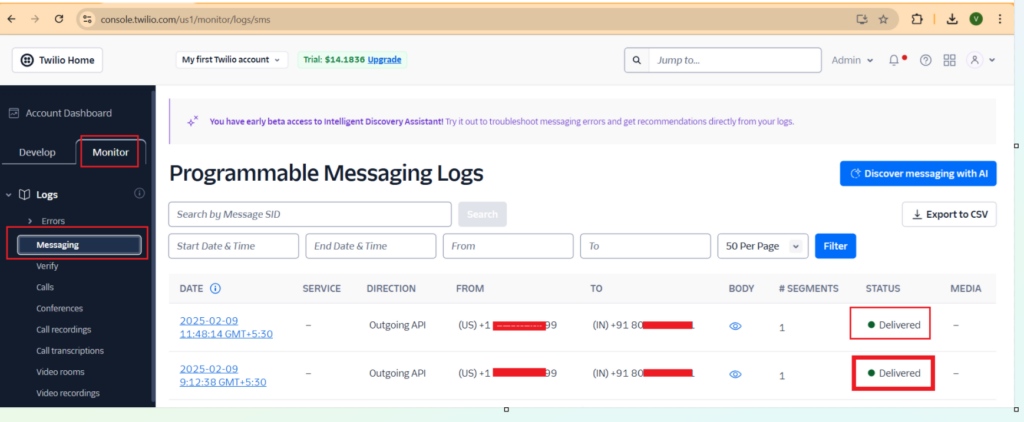
Using Programmatic way
So we have seen how can we see the status using the Twilio Dashboard. Now we ‘ll see the programmatic way to get the status.
If you remember while we send the SMS, we got the sid as response, We ‘ll use the same sid and create a GET api to fetch the information about the SMS status.
See below image for your reference to remembering about the sid.
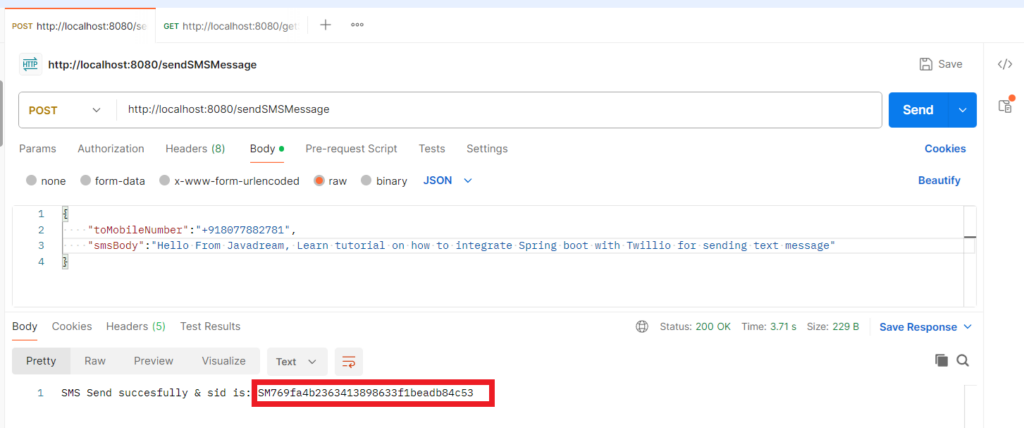
Create a GET api that accept the sid as parameter. Use below code snippet
@GetMapping("/getSMSStatus/{sid}")
public SMSDeliveryStatus getSMSStatus(@PathVariable String sid) {
SMSDeliveryStatus sendSMSStatus = twillioSMSService.getSendSMSStatus(sid);
return sendSMSStatus;
}
Code language: JavaScript (javascript)
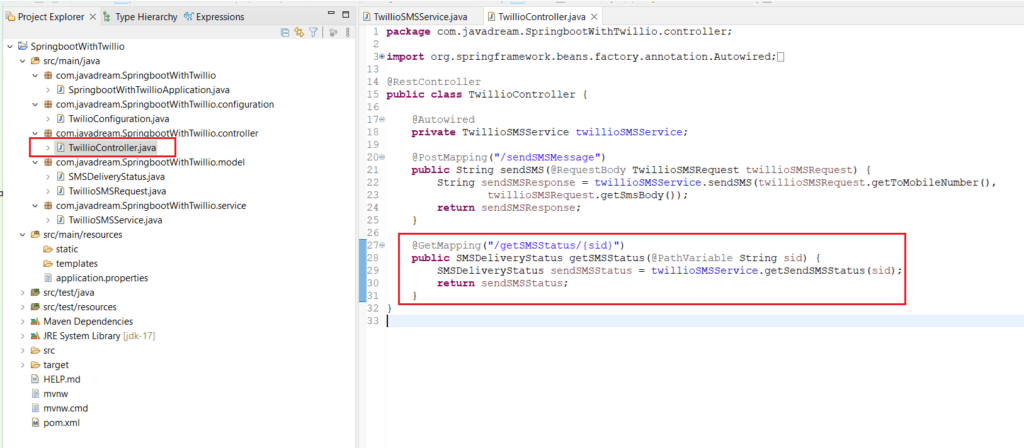
Create a POJO class that is used to return a json response with all the details of SMS status. We created class with name SMSDeliveryStatus.
package com.javadream.SpringbootWithTwillio.model;
public class SMSDeliveryStatus {
private String costToDeliver;
private String currencyCodeOfDeliveryCost;
private String currencyNameOfDeliveryCost;
private String status;
private String toMobileNumber;
private String twillioNumberToSendSMS;
private String messageBody;
public String getCostToDeliver() {
return costToDeliver;
}
public void setCostToDeliver(String costToDeliver) {
this.costToDeliver = costToDeliver;
}
public String getCurrencyCodeOfDeliveryCost() {
return currencyCodeOfDeliveryCost;
}
public void setCurrencyCodeOfDeliveryCost(String currencyCodeOfDeliveryCost) {
this.currencyCodeOfDeliveryCost = currencyCodeOfDeliveryCost;
}
public String getCurrencyNameOfDeliveryCost() {
return currencyNameOfDeliveryCost;
}
public void setCurrencyNameOfDeliveryCost(String currencyNameOfDeliveryCost) {
this.currencyNameOfDeliveryCost = currencyNameOfDeliveryCost;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getToMobileNumber() {
return toMobileNumber;
}
public void setToMobileNumber(String toMobileNumber) {
this.toMobileNumber = toMobileNumber;
}
public String getTwillioNumberToSendSMS() {
return twillioNumberToSendSMS;
}
public void setTwillioNumberToSendSMS(String twillioNumberToSendSMS) {
this.twillioNumberToSendSMS = twillioNumberToSendSMS;
}
public String getMessageBody() {
return messageBody;
}
public void setMessageBody(String messageBody) {
this.messageBody = messageBody;
}
}
Code language: JavaScript (javascript)
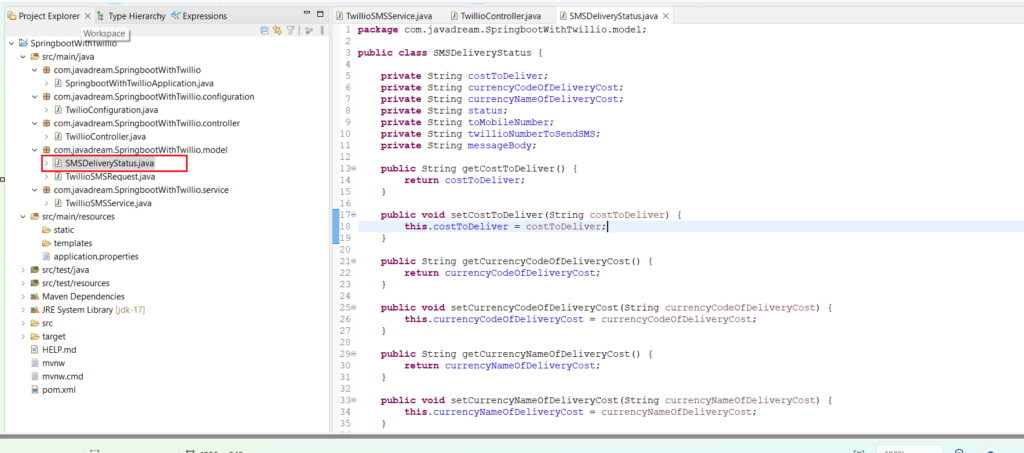
Now go to your service class and add the business logic to get all details about the SMS that we send to the client.
public SMSDeliveryStatus getSendSMSStatus(String sid) {
Message messageStatus = Message.fetcher(sid).fetch();
String price = messageStatus.getPrice();
String currencyCode = messageStatus.getPriceUnit().getCurrencyCode();
String currencyName = messageStatus.getPriceUnit().getDisplayName();
String status = messageStatus.getStatus().name();
String toMobileNumber = messageStatus.getTo();
PhoneNumber twillioMobileNo = messageStatus.getFrom();
String sendSMSText = messageStatus.getBody();
SMSDeliveryStatus deliveryStatus = new SMSDeliveryStatus();
deliveryStatus.setCostToDeliver(price);
deliveryStatus.setCurrencyCodeOfDeliveryCost(currencyCode);
deliveryStatus.setCurrencyNameOfDeliveryCost(currencyName);
deliveryStatus.setStatus(status);
deliveryStatus.setToMobileNumber(toMobileNumber);
deliveryStatus.setTwillioNumberToSendSMS(twillioMobileNo.toString());
deliveryStatus.setMessageBody(sendSMSText);
return deliveryStatus;
}
Code language: JavaScript (javascript)
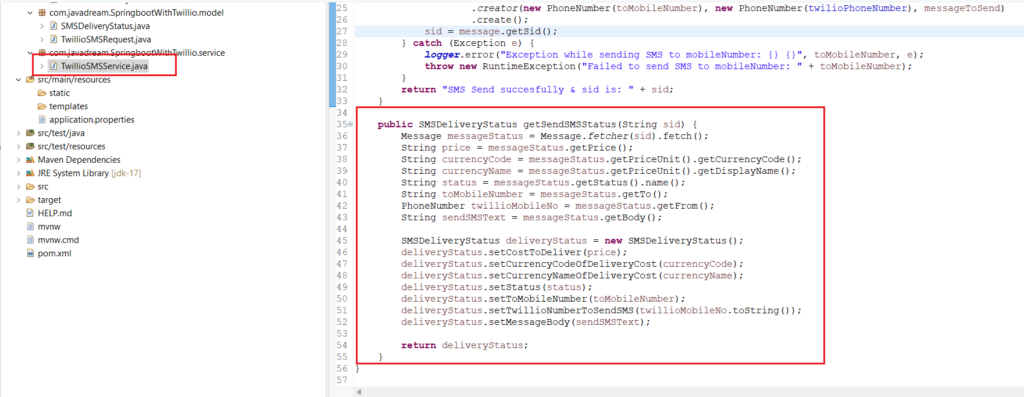
Now open the postman application. And call this GET api to get the status information about the SMS that we send to the client.
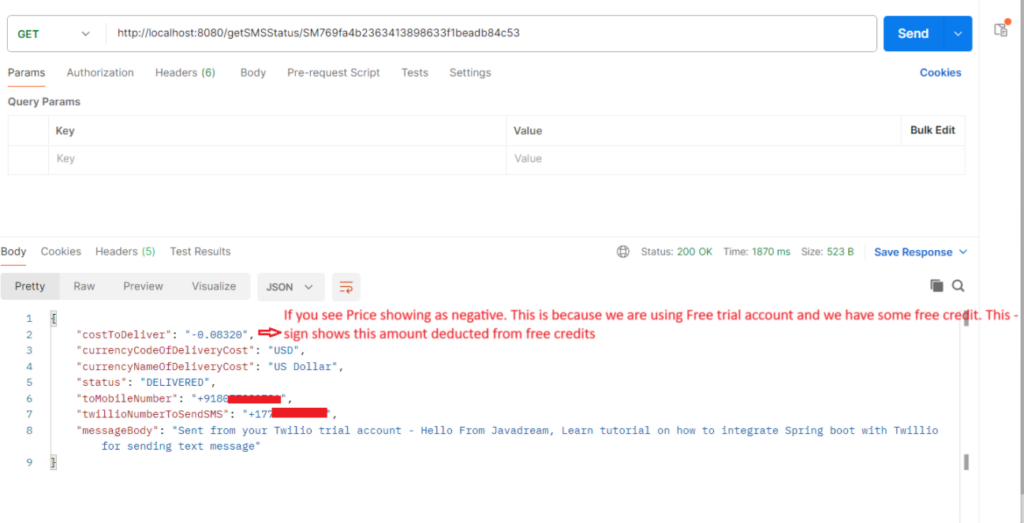
Reference
Follow Twilio official docs on https://www.twilio.com/en-us/blog/send-basic-sms-java-springboot