Spring boot H2 database is the in-memory database provided by the spring boot. As we know that database is the most important thing while we are working on any web based application for storing and retrieving the information, So instead of creating the setup for database like installing MySQL, MongoDB or any other database, We can use the in-memory database called H2 database provided by the spring boot.
Spring boot H2 database is the in-memory database and it is volatile in nature by default. That means data stored in the database until our application is running.
Let’s see how can we use embedded H2 database in spring boot application. To use H2 database we have to add below maven dependency in our pom.xml file
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
After adding the above maven dependency, We have to add below properties in our application.properties file
#Microservice Name (Note: you can give any name)
spring.application.name=SpringbootH2
#DB configuration for H2 database
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=javadream
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
#enable the H2 DB console and given the custom path for opening the H2 DB
spring.h2.console.enabled=true
spring.h2.console.path=/javadream-db-console
First property is for setting our microservice name. After that we have given our database details along with username and password. This username and password needs to be provided in the H2 console.
Let’s discuss about the last two and important configuration.
By Default H2 database console is disable, So we have to enable it by setting the below property. If we do not add the below property in our application properties file we ‘ll not be able to access the H2 database console after running our application.
spring.h2.console.enabled=true
H2 database by default path is /h2-console, Means if your application is running on port 8080 then to access the H2 console you have to use the below URL.
http://localhost:8080/h2-console
So if you want to change this default path and want to give the custom path as per your choice. So you can set the path with the below property for the H2 database console.
spring.h2.console.path=/javadream-db-console
After setting the above property now your H2 database console ‘ll be accessible on the below path.
http://localhost:8080/javadream-db-console
Now we are done just start your application and try to access the H2 console. If you haven’t set the path of console then you can access the H2 console via http://localhost:8080/h2-console URL but if you have set the custom path then just use the path that you have provided in our case it ‘ll be http://localhost:8080/javadream-db-console
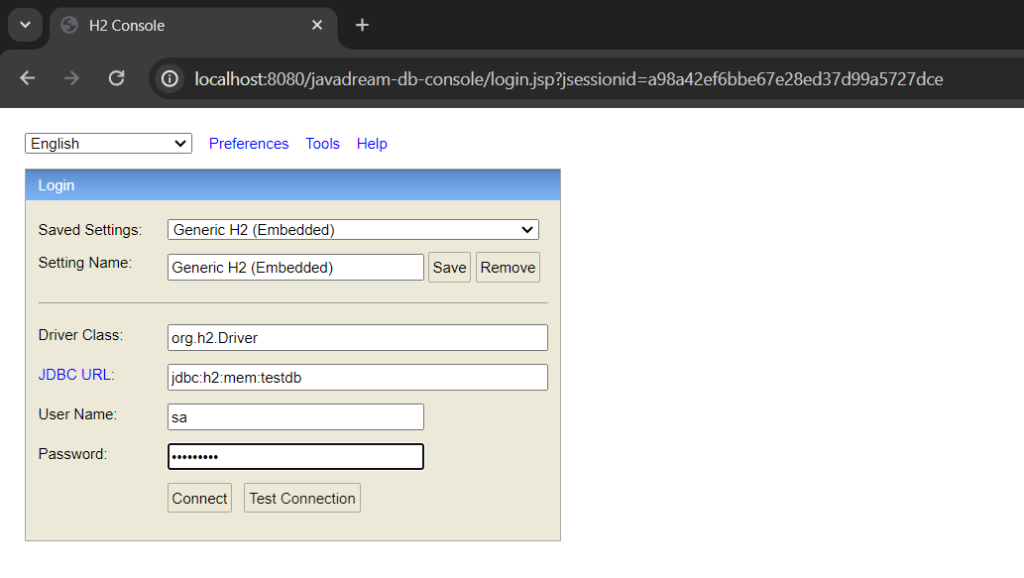
provide the username and password that you given in your application.properties file and click on Connect, You can see the the H2 database console.
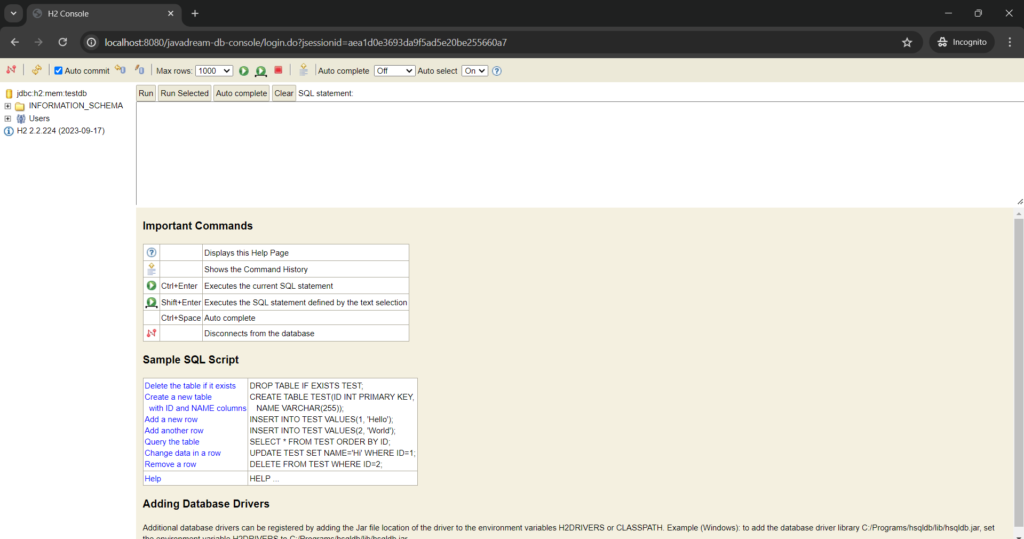