Service Discovery in spring boot act as a database which contains the information about all the running microservices. While using service discovery we don’t need to worry about maintaining the details of the running microservice like what is the URL and port on which a microservice running, and how many instance if a individual microservice running. These all things taken care by service discovery.
Netflix Eureka is used for the service discovery in spring boot. Netflix Eureka implements client-side discovery, Means when any microservice instance start running it ‘ll register itself into the eureka server.
Let’s see how to use Netflix Eureka as a service discovery Server in spring boot.
We have to add the below dependency for eureka server in our pom.xml file
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
After adding the above dependency use the @EnableEurekaServer annotation in your main class.
package com.javadream.EurekaServer;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EurekaServerApplication {
public static void main(String[] args) {
SpringApplication.run(EurekaServerApplication.class, args);
}
}
Now open the application.properties file and put the below configuration there.
spring.application.name=EurekaServer
server.port=8761
eureka.client.registerWithEureka=false
eureka.client.fetchRegistry=false
Port 8761 is the recommended port for the eureka server. It’s not mandatory you can change this port number as per your choice for the server.port property.
We eureka.client.registerWithEureka set property as false. It means it does not need to register itself it should act as a server.
We eureka.client.fetchRegistry set property as false. It means it does not need to fetch registry from others.
That’s all see how easy it is to use netflix eureka. We just have to follow above simple steps. Now run your application and open the URL http://localhost:8761/ . You ‘ll see your eureka server up and running there.
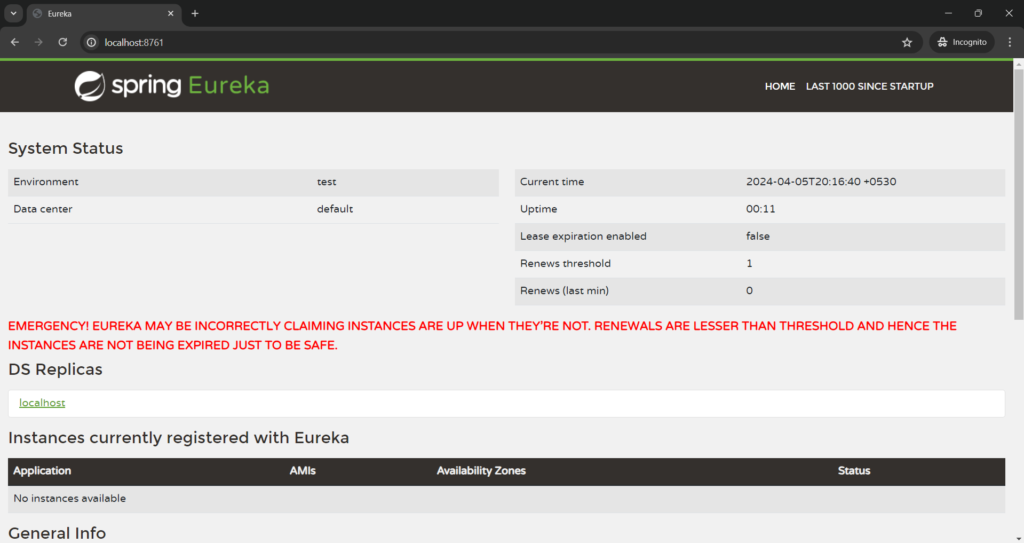